Web Development in Python
Flask - Web Framework for Python
Flask is a lightweight and versatile
web framework for Python. It is developed by Armin Ronacher. It is designed to
be simple, easy to use. It provides the essentials required for web
development. Flask is flexible and it allows developers to make choices regarding
components they want to use and how they want to structure their application.
A web development framework is a set of
pre-written code and tools. It simplifies the process of building and deploying
web applications. It provides a structured and organized way to develop web
applications by offering a set of libraries, conventions, and guidelines.
Flask is a popular and widely used web
framework for Python. It is a lightweight and flexible framework that makes it
easy to get started with web development. The features of Flask are as under:
Routing:
Flask allows developers to define URL patterns and associate them with specific
functions, making it easy to handle different HTTP requests.
Templates:
It includes a Jinja2 templating engine, enabling the dynamic rendering of HTML
templates. This makes it straightforward to create dynamic web pages.
Werkzeug
Integration: Flask builds on the Werkzeug WSGI (Web Server
Gateway Interface) toolkit. It provides a set of utilities for web
applications, including request and response handling.
Extensibility:
Flask follows a "micro" framework philosophy, providing only the
essentials, but it can be extended with various Flask extensions for added
functionality, such as database integration, authentication, and more.
Development
Server: Flask comes with a built-in development server, making
it easy to test and debug applications during development.
Jinja2:
Jinja2 is a popular templating engine for Python. It is widely used in web development frameworks such as Flask and Django. Jinja2 allows you to embed dynamic content within templates, making it easier to generate HTML, XML, or any other mark-up language.
Jinja2 templates are a powerful tool for creating dynamic content in web applications. They provide a clean and readable way to mix static and dynamic elements in your HTML or other mark-up languages.
Variable Substitution:
The developer can use double curly braces {{ ... }} to insert variables into templates.
<p>Hello,
{{ user }}!</p>
Control
Structures:
Jinja2 or Jinja3 supports control structures such as if, for, and block. For example:
{% for i in range(1, 11) %}
<tr>
<td>{{ number }}</td>
<td>x</td>
<td>{{i}}</td>
<td>=</td>
<td>{{ number*i }}</td>
</tr>
{% endfor %}
Virtual Environment
A
virtual environment in the context of Flask, or any Python project, plays a
crucial role in managing project dependencies, isolating packages, and ensuring
a consistent environment across different projects.
Isolation of Dependencies:
A
virtual environment creates an isolated environment for the project under
development. It means that the Python interpreter and installed packages are
specific to the project.
Dependency Management:
Flask applications often rely on specific versions of packages or libraries. By using a virtual environment, the developer can define and manage these dependencies in a requirements.txt file.
Environment Consistency:
Virtual
environments help maintain a consistent development and deployment environment.
The developed and tested code when shared with others or deployed on to a
server, the virtual environment ensures that the required packages are
installed, preventing compatibility issues.
Directory Structure of Website created using Flask:
flask_web_app/
|--
venv/ -It contains the virtual environment for developed project
|--
app/ - It contains the application logic, templates, and static files
| |-- static/ - It stores static files such as
CSS, JavaScript, images, etc.
| |
|-- style.css
| |-- templates/ - This directory contains
HTML templates that are rendered by Flask.
| |
|-- index.html
| |-- __init__.py – It makes the app directory
a Python package, allowing it to be imported as
a module.
| |-- routes.py - It defines how different
URLs should be handled.
|--
config.py – It contains configuration settings for the Flask application.
|--
requirements.txt – It is used to recreate the virtual environment on another
system.
|--
run.py - It typically includes code to create the Flask app instance and start
the
development server.
Developing Dynamic and Interactive Website in Python
A Website is a collection of Webpages. It is accessed through the internet. A website is based on Client-Server model. A Web page is either a static page or a dynamic page. The attached video demonstrates the development of an interactive and dynamic website of two pages using flask framework for Web development in Python.
A static webpage in HTML accepts a
number using HTML form and validation is performed using client-side script. A
dynamic webpage is created using jinja2 templates that allows to embed dynamic content
within templates to generate the table of the entered number. The code for index.html is as under:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Number Table Generator</title>
</head>
<body>
<h1>Welcome to Dynamic and Interactive Website!!!</h1>
<h3>using Flask Web Development Framework</h3>
<form action="/generate_table" method="post">
<label for="accepted_number">Enter a number:</label>
<input type="number" id="accepted_number" name="accepted_number" required>
<button type="submit">Generate Table</button>
</form>
</body>
</html>
Dynamic Web Page:
or data input. The content may be personalized for each user. Here, Jinja2 templating is used to generate the dynamic content for the webpage to display the table of the accepted number. The code for table.html is as under:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Generated Table</title>
</head>
<body>
<h1>Table Generated For: {{number}}</h1>
<table
cellpadding="4"
cellspacing="5">
{% for i in range(1, 11) %}
<tr>
<td>{{ number }}</td>
<td>x</td>
<td>{{i}}</td>
<td>=</td>
<td>{{ number*i }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
Steps to create Flask Framework based Website:
- Open
a terminal or command prompt.
- Navigate
to the project directory.
- Run
the following commands:
# On Windows
python -m venv venv
# On Unix or MacOS
python3 -m venv venv
4. Activate the virtual environment
On Windows: venv\Scripts\activate
On Unix or MacOS:
source venv/bin/activate
5. Install Flask and other dependencies:
pip install Flask
app/__init__.py:
with comments explaining its purpose:
#imports the Flask class from the Flask module. It is necessary to create a Flask web application.
from flask import Flask, render_template
#Create Flask App Instance.
#The __name__ argument is used to determine the root path of the application.
app = Flask(__name__)
from app import routes
| |-- routes.py -
It defines how different URLs should be handled. The code defines to functions index() and generate_table() for the static and the dynamic page. This function is associated with the root URL. When a user accesses the root URL, Flask calls index function, and it rendered the index.html page. The code with comments for routes.py is as under:
from flask import render_template, request
from app import app
#The route() function of the Flask class is a decorator.It tells the application which URL should call the associated function.
#app.route(rule, options)
#The rule parameter represents URL binding with the function.
#The options is a list of parameters to be forwarded to the underlying Rule object.
#It is a decorator that defines a route for the root URL '/'.
#In Flask, routes are used to map URLs to functions. In this case, the function that follows
@app.route('/')
def index():
return render_template('index.html')
@app.route('/generate_table', methods=['POST'])
def generate_table():
accepted_number = int(request.form['accepted_number'])
return render_template('table.html', number = accepted_number)
|-- run.py -
It typically includes code to create the Flask app instance and start the development server. The code with comments is as under:
from app import app
if __name__ == '__main__':
app.run(debug=True)
#run() is used to start the application
#It enables debug support
#The server will then reload itself if the code changes.
#It will also provide a useful debugger to track the errors if any, in the application.
Run & Test Flask Application
To run this Flask application, one can execute the script (python app.py) in the terminal or command prompt. After starting the development server, One can visit http://127.0.0.1:5000/ in web browser where index.html page is rendered.
Web Development Frameworks in Python
The
other popular web development frameworks in Python other than Flask. Each has
its strengths, use cases, and features.
Django:
Django
is a high-level, full-stack web framework that encourages rapid development and
clean, pragmatic design. It follows the "don't repeat yourself" (DRY)
principle.
Key Features:
- ORM (Object-Relational Mapping) for database interaction.
- Admin interface for automatic CRUD operations.
- Authentication system.
- Built-in template engine.
Pyramid:
Pyramid
is a lightweight and flexible web framework. It allows you to start small and
grow as needed, making it suitable for both small and large applications.
Key Features:
- Modularity and flexibility.
- Supports various templating engines.
- URL dispatching and routing.
CherryPy:
CherryPy
is an object-oriented web framework. It allows developers to build web
applications in a similar way to how they would build any other object-oriented
Python program.
Key Features:
- Built-in web server.
- Simplicity and ease of use.
- Can run multiple HTTP servers simultaneously.
Each framework has its own strengths and is suited for different types of projects. This post focus on flask as a Web application development framework.
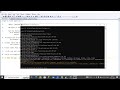
No comments: